If you are reading this post, you probably asked yourself before how server with custom textures (e.g origin realm, tubnet, mcc island) are able to create a ton of new content, from simple items to complex entities, GUI from a simple resource pack. After some research and work, I decided to show you what I discovered and what I created using a resource pack and a basic server π
Table of content
- Finding an idea and creating the model
- Importing in game, the texture id
- Coding some stuff
- Going further
Finding an idea and creating the model
First thing first for creating an item you obviously need an idea of what you want to make. I decided to make a Gravity gun, with this item you would be able to right click entity to move them around and right click them again when you want to drop it. Pretty simple idea but really fun to make. As I wanted to make a gun, I started a new project in BlockBench and made a kind of portal gun here is the result and I’m really happy about it π
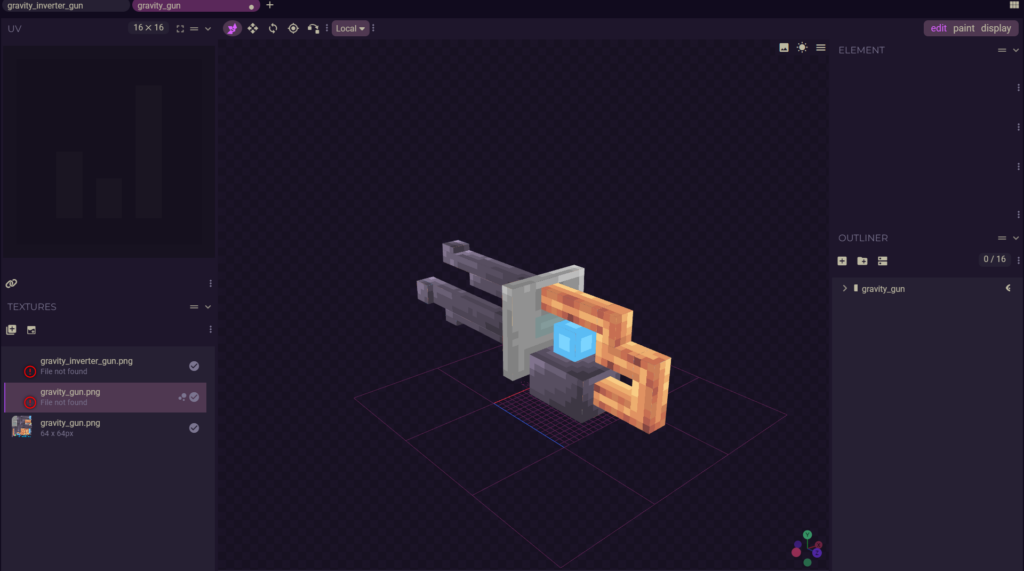
Importing in game, the texture id
Now that we have our model, let’s add it in game ! But wait a second, how can you add an item without replacing one ??? And it’s at that point that you discover the Texture id concept ! The texture id is a hidden value that can be applied to an item so that you can change his texture in a resource pack. To give you an item with a custom id it’s fairly simple you just need to use the following command
/give @s minecraft:paper{CustomModelData:667}
with “paper” being the item and “667” the Custom model data.
Now that we have our item we need to link it in the resource pack for that we will need to create a paper.json file in the models/item/ folder with that code inside
{
"parent": "minecraft:item/generated",
"textures": {
"layer0": "minecraft:item/paper"
},
"overrides": [
{"predicate": {"custom_model_data":667}, "model": "item/gravity_gun"}
]
}
We can see in this json that if the item has a custom_model_data of 667 the model will be our gravity_gun.json in the model/item/ folder. So after implementing these files I had this folder structure for my resource pack.
assets/
ββ minecraft/
β ββ models/
β β ββ item/
β β β ββ paper.json
β β β ββ gravity_gun.json
β ββ textures/
β β ββ item/
β β β ββ gravity_gun.png
pack.mcmeta
pack.png
And here is the resource pack :
Coding some stuff
For the coding I’m not going to go in too much details but essentially I used a spigot server and a plugin that I made. here is how I get the item
package dev.ayabusa.gravitygun.items;
import org.bukkit.Material;
import org.bukkit.inventory.ItemStack;
import org.bukkit.inventory.meta.ItemMeta;
public class GravityGunItem{
public ItemStack getGravityGun() {
// create new paper item
ItemStack item = new ItemStack(Material.PAPER);
// create a new meta for our item
ItemMeta meta = item.getItemMeta();
// set the CustomModelData to 667
meta.setCustomModelData(667);
// change the name of the item
meta.setDisplayName("Β§dGravity Gun");
// and finnaly apply the item meta to the item
item.setItemMeta(meta);
// return the item with his meta
return item;
}
}
And here is the code to move the mob around (spaghetti code I know but it works, sooooo).
// this the event located in my event handler
@EventHandler
public void onPlayerInteractEntity(PlayerInteractEntityEvent event) {
// this to prevent firing two times the event as bukkit does it for each hand
if(event.getHand()==EquipmentSlot.OFF_HAND) {return;}
// get essential variable
Player player = event.getPlayer();
Entity target = event.getRightClicked();
// get the item from the previous code snippet
ItemStack item = new GravityGunItem().getGravityGun();
// return if no item in hand or no CustomModelData
if(player.getInventory().getItemInMainHand()==null || !player.getInventory().getItemInMainHand().hasItemMeta() || !player.getInventory().getItemInMainHand().getItemMeta().hasCustomModelData()) {return;}
// check if the item is a paper with CustomModelData 667
if(player.getInventory().getItemInMainHand().getItemMeta().getCustomModelData()==667 && player.getInventory().getItemInMainHand().getType() == Material.PAPER) {
// I forgot what this does... but I think it's to check if the player is already using the gun
int index = 0;
while (index < playerUsingGG.size()) {
if(playerUsingGG.get(index) == player) {
System.out.println("already using it");
playerUsingGG.remove(index);
return;
}
index = index + 1;
}
playerUsingGG.add(player);
// get the distance between the player and the target
distance = player.getLocation().distance(target.getLocation());
// we don't want our mob to fall so no gravity
target.setGravity(false);
// wait did I really used thread ?! don't rember how this work sorry
Thread newThread = new Thread(() -> {
while(playerUsingGG.contains(player)) {
// does the math thingy
Location loc = player.getLocation();
Vector direction = loc.getDirection().normalize();
double x = direction.getX()*distance;
double y = direction.getY()*distance+1.5;
double z = direction.getZ()*distance;
loc.add(x,y,z);
// tp the mob to the location
target.teleport(loc);
}
// we add gravity back to our target
target.setGravity(true);
// to prevent our mob from going insane with velocity
Vector velocity = new Vector(0,0,0);
target.setVelocity(velocity);
});
// start the thread
newThread.start();
}
else {
// debug stuff
player.getInventory().addItem(item);
}
return;
}
And if you want to test the plugin you can download it here :
Here is the result ! (Sorry for the bad quality, wordpress didn’t want to let me upload a bigger file D: )
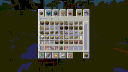
Going further
Great ! We have created our item. But in term of custom content using resource pack it’s just the tip of the iceberg. So if you want to explore a little bit more I recommend you checking some server, and using a mod like ServerPackUnlocker to see how things are made and how to do it yourself. Btw if you want to create custom mobs I recommend checking out Model engine, I never used it but it seems to be the best way to make custom mobs π€©οΈ
See you next time !
– Ayabusa